Featured
- Get link
- Other Apps
WHAT IS A SD-CARD MODULE AND HOW TO USE IT?
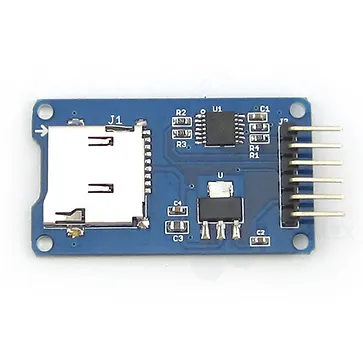
- CS-pin to pin no. 4 on Arduino.
- SCK- pin to pin no.13 of Arduino.
- MOSI pin to pin no. 11 of Arduino.
- MISO pin to pin no. 12 of Arduino.
- VCC pin to 5v and GND to GND.
- CS-pin to pin no. 10 on Arduino.
- SCK- pin to ICSP pin of Arduino.
- MOSI pin to ICSP pin of Arduino.
- MISO pin to ICSP pin with resistance of 330 ohms to Arduino.
- VCC pin to 5v and GND to GND.
#include <SPI.h>
int cs = 4;
File file;
void setup()
{
Serial.begin(9600);
initializeSD();
createFile("test.txt");
writeToFile("This is sample text!");
closeFile();
openFile("prefs.txt");
Serial.println(readLine());
Serial.println(readLine());
closeFile();
}
void loop()
{
}
{
Serial.println("Initializing SD card...");
pinMode(cs, OUTPUT);
{
Serial.println("SD card is ready to use.");
} else
{
Serial.println("SD card initialization failed");
return;
}
}
{
file = SD.open(filename, FILE_WRITE);
{
Serial.println("File created successfully.");
return 1;
} else
{
Serial.println("Error while creating file.");
return 0;
}
}
{
if (file)
{
file.println(text);
Serial.println("Writing to file: ");
Serial.println(text);
return 1;
} else
{
Serial.println("Couldn't write to file");
return 0;
}
}
{
if (file)
{
file.close();
Serial.println("File closed");
}
}
{
file = SD.open(filename);
if (file)
{
Serial.println("File opened with success!");
return 1;
} else
{
Serial.println("Error opening file...");
return 0;
}
}
{
String received = "";
char ch;
while (file.available())
{
ch = file.read();
if (ch == '\n')
{
return String(received);
}
else
{
received += ch;
}
}
return "";
}
#include <SD.h> //include SD card module library
#include <TMRpcm.h> //include speaker library
#define SD_CSP 10 //define CS pin
TMRpcm tmrpcm; //crete an object for speaker library
void setup()
{
tmrpcm.speakerPin = 9; //define speaker pin
if (!SD.begin(SD_CSP))
{ //see if the card is present and can be initialized
return;
}
tmrpcm.setVolume(5.95); //0 to 7. Set volume(Keeping it kind of low works well because its only 8 bit audio)
Serial.begin(9600);
}
void loop()
{
if(Serial.available()>0)
{
int i= Serial.read();
if(i=='1')
{
tmrpcm.play("1.wav"); //the wav file'1' plays
}
if(i=='2')
{
tmrpcm.play("2.wav"); //the wav file'2' plays
}
if(i=='3')
{
tmrpcm.play("3.wav"); //the wav file'3' plays
}
if(i=='4')
{
tmrpcm.play("4.wav"); //the wav file'4' plays
}
if(i=='5')
{
tmrpcm.play("5.wav"); //the wav file'5' plays
}
}
}
#include <SD.h> // need to include the SD library
//#define SD_ChipSelectPin 53 //example uses hardware SS pin 53 on Mega2560
#define SD_ChipSelectPin 4 //using digital pin 4 on arduino nano 328, can use other pins
#include <TMRpcm.h> // also need to include this library...
#include <SPI.h>
TMRpcm tmrpcm; // create an object for use in this sketch
void setup(){
tmrpcm.speakerPin = 9; //5,6,11 or 46 on Mega, 9 on Uno, Nano, etc
Serial.begin(9600);
if (!SD.begin(SD_ChipSelectPin)) // see if the card is present and can be initialized:
{
Serial.println("SD fail");
return; // don't do anything more if not
}
tmrpcm.play("music"); //the sound file "music" will play each time the arduino powers up, or is reset
}
void loop()
{
if(Serial.available())
{
if(Serial.read() == 'p'){ //send the letter p over the serial monitor to start playback
tmrpcm.play("music");
}
}
}
- Get link
- Other Apps
Popular Posts
PIR SENSOR, EVERYTHING A BEGINNER NEEDS TO KNOW.
- Get link
- Other Apps
Comments
Post a Comment